Merge Tags
Merge tags are placeholders in an email template that automatically insert personalized or dynamic content, such as a recipient's name, unsubscribe link, or custom data.
They follow a specific format and are replaced with real values when the email is sent. This helps create more personalized and engaging emails without manual input. Default format of merge tags is:
*|MERGE_TAG|*
Defining Merge Tags
Topol Plugin is pre-defined with only one merge tag for dynamic insertion of customer's first name:
*|FIRST_NAME|*
To dynamically insert additional values into your template, such as a customer's full name, academic title, email, or personalized greeting, you need to define your custom merge tags in a mergeTags
array within the default TOPOL_OPTIONS
object using the syntax shown in this example:
mergeTags: [
{
name: "Name tags", // Group of merge tags
items: [
{
value: "*|FIRST_NAME|*", // Text to be inserted
text: "First name", // Shown text in the menu
label: "Customer's first name", // Shown description title in the menu
},
{
value: "*|LAST_NAME|*",
text: "Last name",
label: "Customer's last name",
},
]
},
name: "Personal details", // Group of merge tags
items: [
{
value: "*|AGE|*",
text: "Age",
label: "Customer's age",
},
{
value: "*|TITLE|*",
text: "Academic title",
label: "Customer's academic title",
},
],
{
name: "Special links", // Group of merge tags
items: [
{
value: '<a href="*|UNSUBSCRIBE_LINK|*">Unsubscribe</a>',
text: "Unsubscribe",
label: "Unsubscribe link",
},
{
value: '<a href="*|WEB_VERSION_LINK|*">Web version</a>',
text: "Web version",
label: "Web version link",
},
],
},
{
name: "Special content", // Group of merge tags
items: [
{
value: 'For more details, please visit our <a href="https://www.shop.shop">e-shop</a>!',
text: "Visit our site",
label: "Call to Action",
},
],
},
]
INFO
It is important to note that, by default, only the merge tags defined within this unique mergeTags
array will be available in the plugin text editor (TinyMCE). However, you can dynamically update all merge tags with new values without needing to rewrite the mergeTags
code each time.
Additionally, you can create custom mergeTags
arrays for different purposes and switch between them dynamically.
The feature that enables you to do all of these things is called Update Merge Tags, which we will discuss in a separate topic at the end of this chapter.
Once defined, you will find them in the text editor toolbar. The following image shows the merge tags organized into a nested structure, which we will discuss next.

Nested Merge Tags
In order to create more complex organization of your merge tags, you can nest groups of merge tags within other groups.
Code example:
mergeTags: [
{
name: "Merge tags", // Main group name
items: [
{
value: "*|FIRST_NAME|*", // Text to be inserted
text: "First name", // Shown text in the menu
label: "Customer's first name", // Shown description title in the menu
},
{
value: "*|LAST_NAME|*",
text: "Last name",
label: "Customer's last name",
},
{
name: "Merge tags nested", // Nested group name
items: [
{
value: "*|FIRST_NAME_NESTED|*", // Text to be inserted
text: "First name 2", // Shown text in the menu
label: "Customer's first name 2", // Shown description title in the menu
},
{
value: "*|LAST_NAME_NESTED|*",
text: "Last name 2",
label: "Customer's last name 2",
},
],
},
],
},
];
Smart Merge Tags
By default, merge tags inserted into templates are static, meaning that once you insert one into your template, you cannot quickly swap it for another merge tag.
To enable this feature and make merge tags easier to use, you can set all merge tags as smart merge tags. Your merge tags will then appear as shown here:
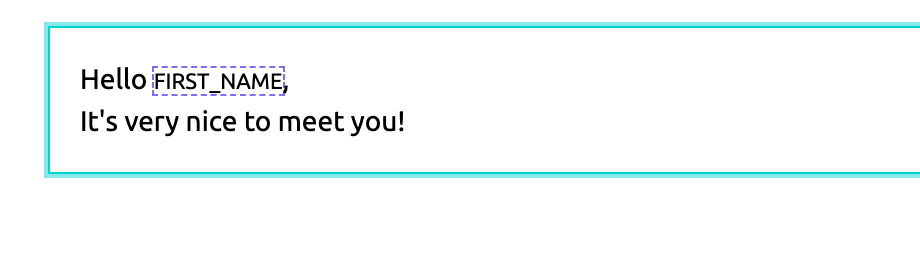
This option allows you to interact with each inserted merge tag by clicking on it. When you do so, a modal window will pop up, allowing you to browse through your other defined merge tags and choose which one will replace the original one in your template.
You can enable the Smart Merge Tags feature by:
smartMergeTags: {
enabled: true,
}
Merge Tag value autocompletion
To avoid constantly browsing through your collection of merge tags, the Topol Plugin offers an autocomplete feature. Just start typing the value of the desired merge tag (remember to use proper syntax), and the autocomplete feature will activate, providing a list of relevant suggestions.
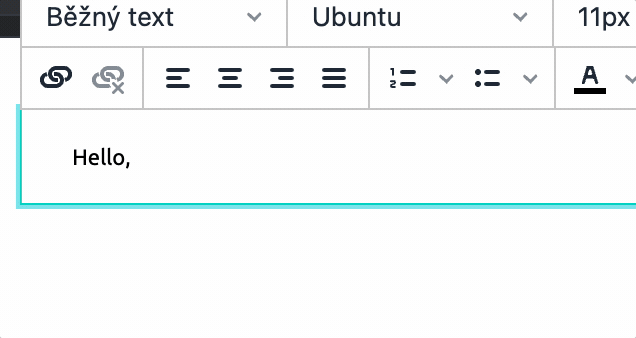
The autocomplete feature is also implemented in many other text fields.
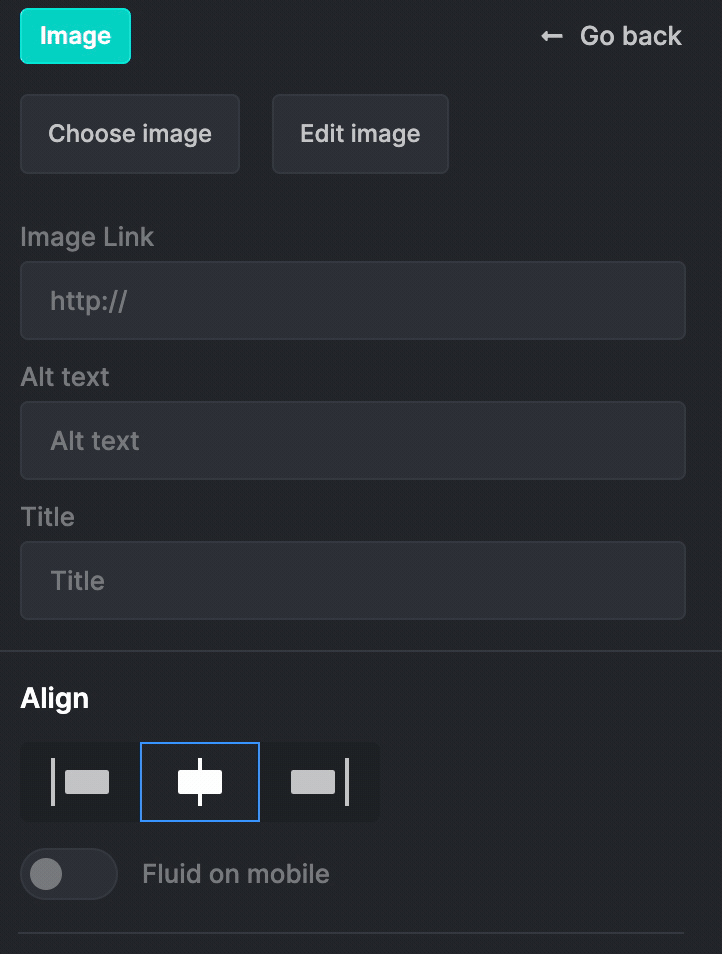
Custom merge tag syntax
In addition to customizing the merge tag values, you can also modify the syntax. If you prefer a syntax different from the default, you can set it up using the following code:
smartMergeTags: {
enabled: true,
syntax: {
start: "{{",
end: "}}"
}
}
In this case, the syntax for your merge tag will be as follows:
{{MERGE_TAG}}
Update Merge Tags
Until now, we have only used the default mergeTags
array, as described here. This predefined set of merge tags was loaded into our plugin at initialization, and changing these tags required rewriting the mergeTags
code.
To make things even more cumbersome, there was no way to switch between different mergeTags
arrays "on the fly" when certain conditions were met (event trigger, change of display mode, etc.).
To fix these issues, you can utilize this callable function:
window.TopolPlugin.setMergeTags(mergeTags);
First, define your custom mergeTags
arrays with standard structure, as shown on the examples below:
const catMergeTags = [
{
name: "Cat Merge Tags",
items: [
{
value: "*|CAT_NAME|*",
text: "Cat Name",
label: "Name of customer's cat",
},
{
value: "*|CAT_BREED|*",
text: "Cat Breed",
label: "Breed of customer's cat",
},
],
},
]
const dogMergeTags = [
{
name: "Dog Merge Tags",
items: [
{
value: "*|DOG_NAME|*",
text: "Dog Name",
label: "Name of customer's dog",
},
{
value: "*|DOG_BREED|*",
text: "Dog Breed",
label: "Breed of customer's dog",
},
],
},
]
In order to dynamically load either of these additional mergeTags
array while the Plugin is running, you have two options:
- Define an event handler that invokes the
window.TopolPlugin.setMergeTags(...)
function with the variable name of the specific merge tags array used as its argument when the event is triggered:
window.TopolPlugin.setMergeTags(catMergeTags);
// or
window.TopolPlugin.setMergeTags(dogMergeTags);
- Run the function in the console within the same browser window where the plugin is already initialized and running. In this case, it's easier to use the array directly as an argument instead of calling it via a variable (option 1). If you still prefer to use the array's variable name, you must also copy its definition to the console (option 2).
// Run in the console within the same browser window where the Plugin is initialized.
// Option 1
window.TopolPlugin.setMergeTags([
{
name: "Cat Merge Tags",
items: [
{
value: "*|CAT_NAME|*",
text: "Cat Name",
label: "Name of customer's cat",
},
{
value: "*|CAT_BREED|*",
text: "Cat Breed",
label: "Breed of customer's cat",
},
],
},
]);
// or Option 2
const catMergeTags = [
{
name: "Cat Merge Tags",
items: [
{
value: "*|CAT_NAME|*",
text: "Cat Name",
label: "Name of customer's cat",
},
{
value: "*|CAT_BREED|*",
text: "Cat Breed",
label: "Breed of customer's cat",
},
],
},
]
window.TopolPlugin.setMergeTags(catMergeTags);
Why can't this function be called during initialization?
You might wonder, "Wouldn't it be easier to call this function when the plugin initializes so that it loads directly with the new merge tags?" This approach would allow us to skip loading the plugin with default merge tags first and only then replacing them with our custom ones.
In theory, this could be done by calling the function right after the plugin initializes, like this:
const TOPOL_OPTIONS = {
// ...
}
TopolPlugin.init(TOPOL_OPTIONS); // Initialize plugin
window.TopolPlugin.setMergeTags(catMergeTags); // Immediately load custom merge tags
When we run this code, the plugin functions as expected, but it still loads with the default merge tags instead of our catMergeTags
array. This happens because the TopolPlugin is not initialized immediately (it loads asynchronously).
Therefore, at the time of executing setMergeTags(catMergeTags)
, the TopolPlugin has not yet loaded, and this function does not exist. Later, when the plugin finally loads with the default merge tags, the call to setMergeTags(catMergeTags)
has already occurred and is not executed again, so the default merge tags are not replaced.
A comprehensive list of all callable functions available in the Topol Plugin can be found here.