Using Custom Top Bar With Topol Plugin
When integrating Topol into other platforms, one of the most frequently requested tasks is to utilize a custom top bar and incorporate existing branded buttons for a seamless user experience.
Topol provides a comprehensive solution to accomplish this task seamlessly. Let's dive right in!
Removing Top Bar from the editor
Let's start with removing the existing top bar completely. We can achieve this by adding removeTopBar: true
to TopolOptions.
After completing this step, the TopolOptions will be transformed into the following configuration:
const TopolOptions = {
id: "#app",
authorize: {
apiKey: "YOUR_API_KEY",
userId: "YOUR_USER_ID",
},
removeTopBar: true,
};
Use custom buttons to manually perform the actions
When using custom buttons we can call window.TopolPlugin
instance to call editor's methods.
For example, if we want to use save manually, we do window.TopolPlugin.save()
. When we call the method editor automatically calls save and returns onSave
callback with html
and json
.
We receive this onSave callback in callbacks section inside TopolOptions:
const TopolOptions = {
id: "#app",
authorize: {
apiKey: "YOUR_API_KEY",
userId: "YOUR_USER_ID",
},
removeTopBar: true,
callbacks: {
onSave(hmtl, json) {
// Save html and json to your database
},
},
};
To better illustrate, let's look at the diagram bellow:
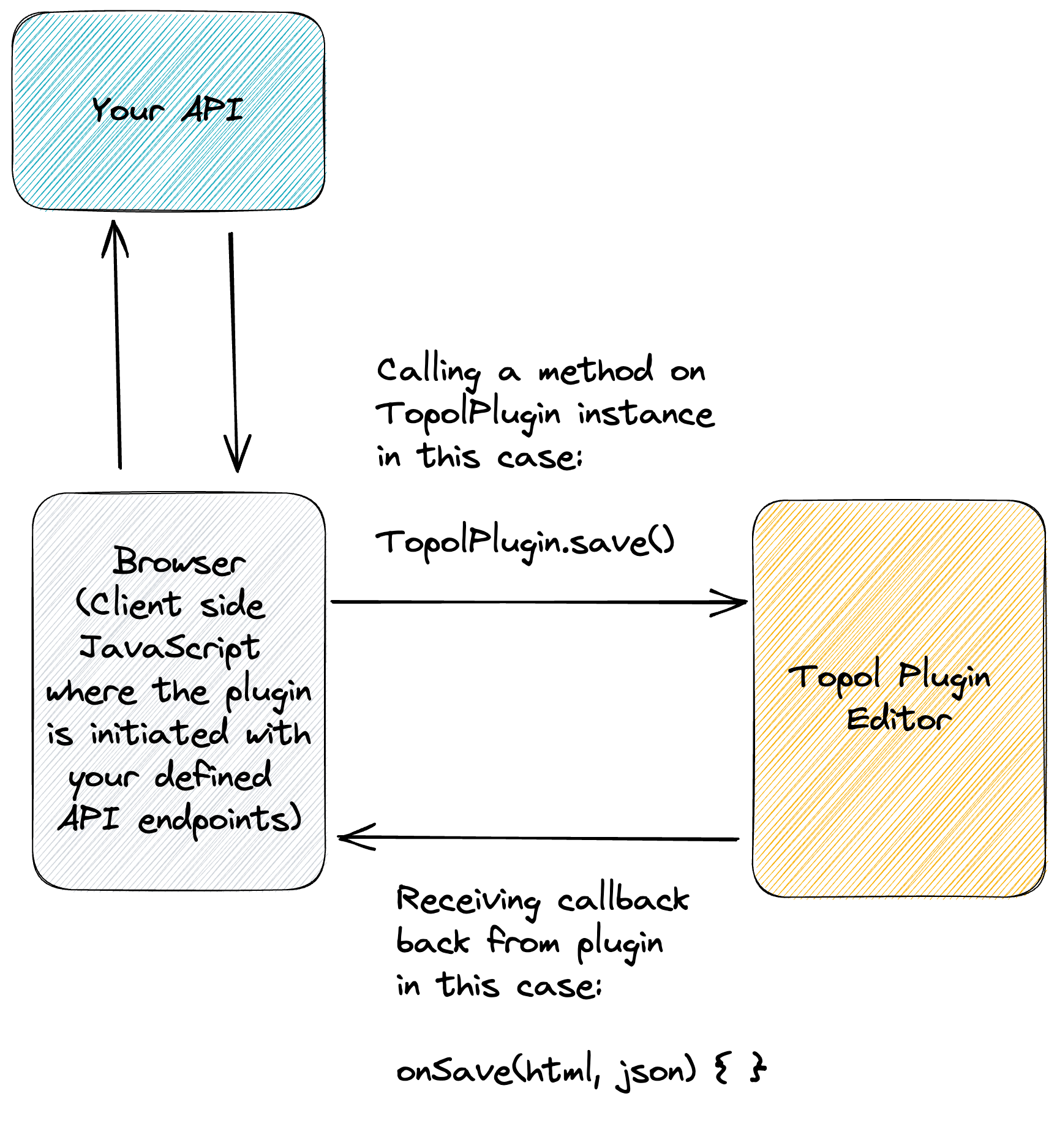
All actions we can implement
In the example above, we showcased saving functionality, but that's only one action Top bar is responsible for other actions that we can implement:
Save and SaveAndClose
We call save method on TopolPlugin instance: window.TopolPlugin.save()
. In the callbacks
const TopolOptions = {
id: "#app",
authorize: {
apiKey: "YOUR_API_KEY",
userId: "YOUR_USER_ID",
},
removeTopBar: true,
callbacks: {
onSave(html, json) {
// Save html and json to your database
},
onSaveAndClose(html, json) {
// Save html and json to your database
// Redirect from editor
},
},
};
Undo and Redo
We call redo method on TopolPlugin instance: window.TopolPlugin.redo()
and undo window.TopolPlugin.undo()
. In the callbacks
const TopolOptions = {
id: "#app",
authorize: {
apiKey: "YOUR_API_KEY",
userId: "YOUR_USER_ID",
},
removeTopBar: true,
callbacks: {
onUndoChange(count) {
// count with number of undo changes
},
onRedoChange(count) {
// count with number of redo changes
},
},
};
Changing preview mode
To change preview mode we call window.TopolPlugin.togglePreview()
to change between mobile and desktop mode inside preview we can use window.TopolPlugin.togglePreviewSize()
Combining with mobile first
On of the features Topol Plugin has is working directly in mobile first environment. You can find more information about this feature here.