How to connect to your API
Certain actions within the various features of the Editor plugin require the use of a custom API. Unfortunately, due to iframe limitations, it is not possible to utilize Promises, which are crucial for achieving the correct behavior.
For better understanding look at the diagram below:
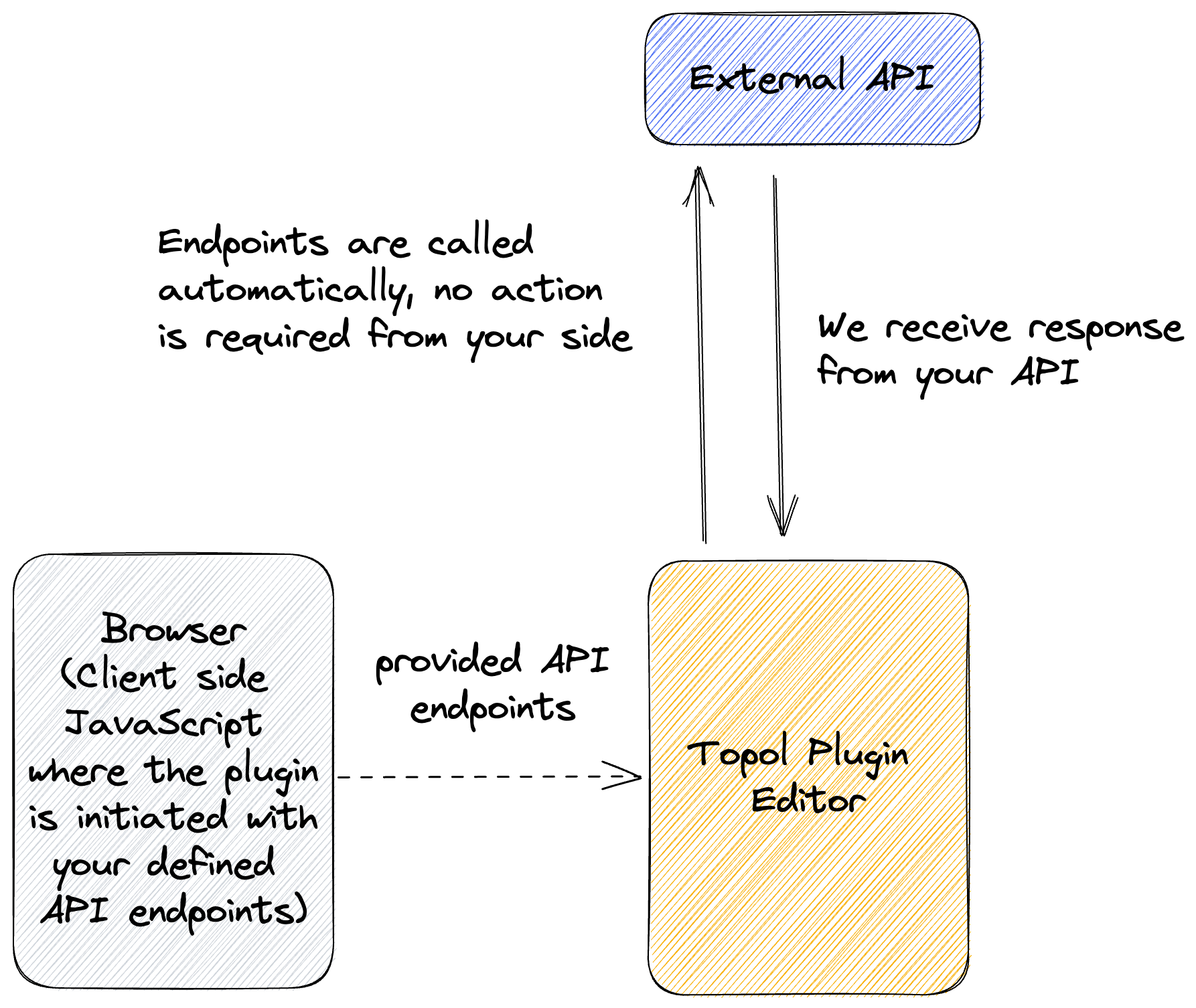
How to enter the API endpoints
API endpoints are added inside TopolOptions as follows, provided URLs are just examples, you can use whatever endpoint name that suits you.
WARNING
When working with custom endpoints don't forget to allow our server d5aoblv5p04cg.cloudfront.net
as a known origin. Otherwise, you are going to run into CORS issues.
const TopolOptions = {
id: "#app",
authorize: {
apiKey: "YOUR_API_KEY",
userId: "YOUR_USER_ID",
},
api: {
// Api endpoints needed for autosaves
GET_AUTOSAVE: "https://your-domain.test/get-autosave",
AUTOSAVES: "https://your-domain.test/autosaves",
AUTOSAVE: "https://your-domain.test/autosave",
// Api endpoints needed for products
FEEDS: "https://your-domain.test/feeds",
PRODUCTS: "https://your-domain.test/products",
// Api endpoints needed for self hosted storage
FOLDERS: "https://your-domain.test/folders",
IMAGE_UPLOAD: "https://your-domain.test/image-upload",
IMAGE_EDITOR_UPLOAD: "https://your-domain.test/image-editor-upload",
},
};
When we use the endpoints we append various names in the end of the provided URL. You can read more specifics in the corresponding sections:
Securing the endpoints
When calling the endpoints you might want to secure the endpoints, so nobody outside your organization can get the data. We allow you to add custom headers to request simply by adding:
const TopolOptions = {
apiAuthorizationHeader: "Bearer token";
};
For more information and andditional options for headers refer to API authentication section